The BAT Cane : A Low-Cost Smart Cane for the Blind
CODING
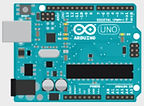
Coding for Arduino Control System
// ==================== TRAVEL CANE PROTOTYPE CODE ==================== \\
/*
-----------------------------------------------------------------------
Jeff Wiest
Human Machine Systems
10/5/2015
-----------------------------------------------------------------------
This Arduino code will detect if the user of a travel cane is:
--> deviating from a straight path
--> approaching an obstacle
These checks are conditional upon the user:
--> turning on switchOne for path deviation
--> turning on switchTwo for obstacle detection
-----------------------------------------------------------------------
*/
// accelerometer sensor setup
int acceleroPin = 5;
int acceleroVal = 0;
// ultrasonic sensor setup
int ultraPin = 4;
int ultraVal = 0;
// vibration motor setup
int vibroPin = 6;
int vibroPWM = 7;
int vibroVal = 0;
// input button setup
int switchOne = 12; // user will toggle switchOne if they want to detect path deviation
int switchTwo = 11; // user will toggle switchTwo if they want to detect obstacles
void setup() {
// initialize Serial
Serial.begin(9600);
// initialize pin modes
pinMode(acceleroPin, INPUT);
pinMode(ultraPin, INPUT);
pinMode(vibroPin, OUTPUT);
pinMode(switchOne, INPUT_PULLUP);
pinMode(switchTwo, INPUT_PULLUP);
}
void loop() {
// check if user wants to sense path deviation
if (digitalRead(switchOne)) {
// read accelerometer
acceleroVal = acceleroVal + acceleroRead();
if (acceleroVal > 5000) { // if deviating right
vibroRight(); // turn on vibrating motor
}
else if (acceleroVal < -5000) { // if deviating left
vibroLeft(); // turn on vibrating motor
}
else { // if no deviation detected
digitalWrite(vibroPin, LOW); // else keep vibrating motor off
}
}
// check if user wants to detect obstacles
if (digitalRead(switchTwo)) {
ultraVal = ultraRead(); // read ultrasonic sensor
if (ultraVal > 700) { // check for obstacle
vibroObstacle(ultraVal); // vibrate motor with intensity proportional to nearness
}
else {
digitalWrite(vibroPin, LOW); // else, keep vibrating motor off
}
}
// run debugger (this line can be commented out to improved speed
displayVals();
} // end void loop
// ==================== ALL THE FUNCTIONS ==================== \\
// accelerometer function
int acceleroRead() {
// read accelerometer values
acceleroVal = analogRead(acceleroPin);
return acceleroVal;
}
// vibrate motor for path deviating right function
void vibroRight() {
// turn on vibrating motor
digitalWrite(vibroPin, HIGH);
}
// vibrate motor for path deviating left function
void vibroLeft() {
// turn on vibrating motor
digitalWrite(vibroPin, HIGH);
}
// ultrasonic function
int ultraRead() {
// read ultrasonic sensor
ultraVal = analogRead(ultraPin);
return ultraVal;
}
// vibrate motor for obstacle detection function
void vibroObstacle(int ultraVal) {
// map ultraVal to PWM scale
ultraVal = map(ultraVal, 0, 1023, 0, 255);
// vibrate motor with intensity from ultraVal
digitalWrite(vibroPin, HIGH);
analogWrite(vibroPWM, ultraVal);
}
// this function will display all pin and output values for debugging purposes
void displayVals() {
// display ALL the values!
Serial.print("accelVal= ");
Serial.print(acceleroVal);
Serial.print("\tultraVal= ");
Serial.print(ultraVal);
Serial.print("\tvibroPWM= ");
Serial.print(vibroPWM);
Serial.print("\tswitchOne= ");
Serial.print(digitalRead(switchOne));
Serial.print("\tswitchTwo= ");
Serial.println(digitalRead(switchTwo));
}
Arduino Official Website
Arduino Open Source Library
Special Thanks, Jeff.